Design · Development · Marketing
Get online and grow fast
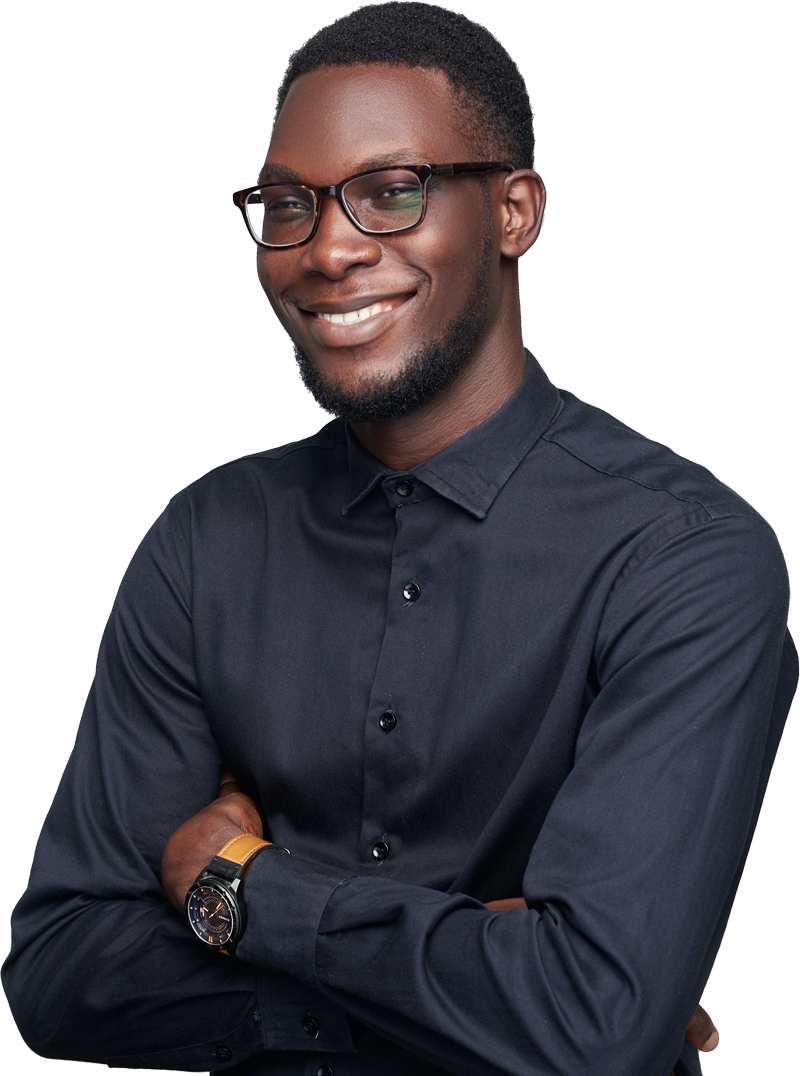
Wooglet Application Store
Wooglet Application Store offers a range of contact options for customers to get support whenever they need it. We are here to maximise customer satisfaction and ensure that our users get a quick resolution to any issues they may have. Here are the various channels through which you can contact Wooglet Application Store: